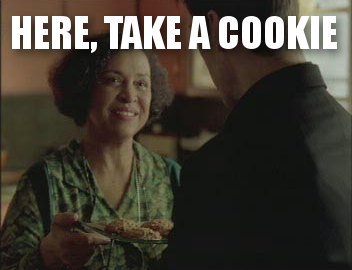
Testing websites using ASP.NET Forms Authentication with Burp Suite
Testing a website is usually considered just another day at work, Burp Suite is usually the tool of our choice for automating some of the scans that apply in this field. Assessing the authenticated part of the site is also common, and since Burp can be used as an HTTP proxy, it can capture our session tokens (usually HTTP cookies) and perform scans just like we’d do as humans. This token is usually remain unchanged over the time of the session, and the session itself is kept alive by the scanner activity itself.
However, a few weeks ago, we encountered an ASP.NET site that offered the regular ASP.NET_SessionId
cookie and after successful login we got another one called .ASPXFORMSAUTH
indicating that the application uses Forms Authentication. We started issuing requests including these two cookies, but within 5 minutes, the server started responding like we’re not logged in. Investigation followed, and we could reproduce that even by sending a request every 10 seconds, after 2 minutes pass from login, the requests with the two original cookies are denied. The operators confirmed that the session timeout is 2 minutes (which is resonable given the normal use-cases of the application), and looking at the responses revealed that around halfway between login and the 2 minutes deadline, a new .ASPXFORMSAUTH
cookie is set by the server.
Apparently, Burp Suite ignored such Set-Cookie headers at the time both in its Scanner and Intruder modules, so I wrote a simple plugin that would hook HTTP requests within Burp and behave like a browser for this specific cookie. If such a cookie is received it gets stored as a protected field of the class, and subsequent requests will be modified to include the latest value. Since Burp uses multiple threads, this value also needs locking in order to avoid race conditions. Burp also offers some help with parsing and mangling HTTP headers, so besides hooking HTTP traffic and initialization, the plugin starts by storing a reference to an instance of the IExtensionHelpers
interface.
public class BurpExtender implements IBurpExtender, IHttpListener
{
protected IExtensionHelpers helpers;
protected String cookie_value = null;
public static final String COOKIE_NAME = ".ASPXFORMSAUTH";
@Override
public void registerExtenderCallbacks(IBurpExtenderCallbacks callbacks)
{
helpers = callbacks.getHelpers();
callbacks.setExtensionName("AspxFormAuth");
callbacks.registerHttpListener(this);
By registering a HTTP listener, the processHttpMessage
method will be called by Burp every time an HTTP request is issued, regardless of its source, including the browser, the Scanner or Intruder modules. The messageIsRequest
parameter can be used to define different behavior for requests and responses; in case of former, any Cookie:
headers with the matching name in the request will be updated to the latest value (if there’s one, hence the null check).
@Override
public void processHttpMessage(int toolFlag, boolean messageIsRequest, IHttpRequestResponse messageInfo)
{
if (messageIsRequest)
{
synchronized (this) {
if (cookie_value == null) return;
}
byte[] request = messageInfo.getRequest();
IRequestInfo ri = helpers.analyzeRequest(request);
List parameters = ri.getParameters();
for (IParameter parameter : parameters) {
if (parameter.getType() == IParameter.PARAM_COOKIE &&
parameter.getName().equals(COOKIE_NAME)) {
synchronized (this) {
messageInfo.setRequest(helpers.updateParameter(request,
helpers.buildParameter(COOKIE_NAME, cookie_value,
IParameter.PARAM_COOKIE)));
}
}
}
}
The only remaining task is feeding the cookie_value member with tokens by parsing responses. Again, the Burp helpers are used to analyze the headers and if a cookie with a matching name is found, its value is stored, taking special care to let only a single thread access the value at a time.
else
{
IResponseInfo ri = helpers.analyzeResponse(messageInfo.getResponse());
List cookies = ri.getCookies();
for (ICookie cookie : cookies) {
if (cookie.getName().equals(COOKIE_NAME)) {
synchronized (this) {
cookie_value = cookie.getValue();
}
}
}
}
Using this technique, we’d been able to perform the assessment, the full source code can be found in a branch of our GitHub repository. If you don’t want to compile it for yourself, a binary JAR is also available on the release page. We’re also proud that since we released the Burp OAuth plugin this one is based on, former has been built upon and improved by SecureIdeas as part of their Co2 plugin.